java GUI 设计
GUI(Graphical User Interfaces):由各种图形对象组成的用户界面,在这种用户界面下,用户的命令和对程序的控制是通过“选择”各种图形对象来实现的。
- 抽象窗口工具包:
- java.awt:提供基本GUI组件,视觉控制,绘图工具等
- java.awt.event:事件处理
- 组件和容器:
布局管理器
- 容器对象.setLayout(布局管理器对象)
- 布局管理器 容器对象.getLayout()
- FlowLayout:流式布局,是Panel(及其子类)默认布局管理器
- 布局效果:组件在容器中按照加入次序逐行定位,行内从左到右,一行排满后换行。组件按原始大小进行显示
-
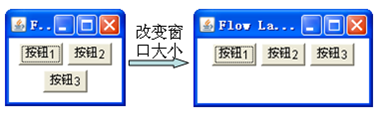
- 构造方法
- public FlowLayout():默认居中对齐
- public FlowLayout(int align):显示设定组件对其方式
- public FlowLayout(int align,int hgap,int vgap):设置水平和垂直间距
- FlowLayout.LEFT左对齐
- FlowLayout.RIGHT 右对齐
- FlowLayout.CENTER居中
import java.awt.*;
public class FlowLayoutDemo{
public static void main(String[] args) {
Frame f = new Frame("流动布局");
Button b1 = new Button("按钮1");
Button b2 = new Button("按钮2");
Button b3 = new Button("按钮3");
f.setLayout(new FlowLayout());
f.add(b1);
f.add(b2);
f.add(b3);
f.setSize(200,300);
f.setVisible(true);
}
}
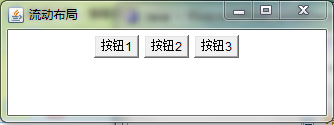
- BorderLayout:边界布局,是Window及其子类类型容器的默认布局管理器。
- 布局效果:将整个容器范围划分成East、West、South、North、Center五个区域,组件只能被添加到指定的区域。
- 在使用边界布局的容器中,组件的尺寸也被布局管理器强行控制,即与其所在区域的尺寸相同。
- 每个区只能加入一个组件,如加入多个,先前的组件会被抛弃
- 当容器的尺寸发生变化时,其中各组件相对位置不变,尺寸随所在区域进行缩放调整;
调整原则:北、南两个区域只能在水平方向缩放(宽度可调),东、西两个区域只能在垂直方向缩放(高度可调),中部区域都可缩放。
- 构造方法
- public BorderLayout()
-
public BorderLayout(int hgap,int vgap) :水平和垂直间距
- 5个区域:
- BorderLayout.EAST
- BorderLayout.WEST
- BorderLayout.SOUTH
- BorderLayout.NOUTH
- BorderLayout.CENTER
-
f.add(btnNorth,"North"); =f.add(BorderLayout.NOUTH);
import java.awt.*;
public class BorderLayoutDemo extends Frame {
Button bNorth,bSouth,bWest,bEast,bCenter;
public BorderLayoutDemo(){
super("边框布局");
bNorth = new Button("按钮1");
bSouth = new Button("按钮2");
bWest = new Button("按钮3");
bEast = new Button("按钮4");
bCenter = new Button("按钮5");
add(bNorth,"North");
add(bSouth,"South");
add(bWest,"West");
add(bEast,"East");
add(bCenter,"Center");
setBounds(200,200,300,300);
setVisible(true);
}
public static void main(String[] args){
new BorderLayoutDemo();
}
}
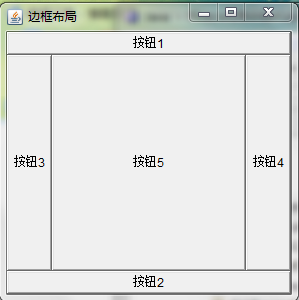
- GridLayout:网格布局
- 布局效果:将容器区域划分成规则的矩形网格,每个单元格区域大小相等。组件被添加到每个单元格中,按组件加入顺序先从左到右填满一行后换行,行间从上到下。
- 行数为设置值,列数则通过指定的行数和布局中的组件总数来进行调整
- 正常情况下使用GridLayout布局时,向容器中加入的组件数目应与容器划分出来的单元格总数相等,但假如出现两者数目不等的情况,程序也不会出错,而是保证行数为设置值,列数则通过指定的行数和布局中的组件总数来进行调整。
-
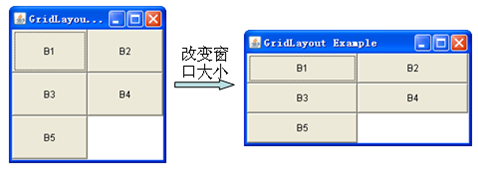
- 构造方法
- public GridLayout()
- public GridLayout(int rows,int cols)
- public GridLayout(int rows,int cols,int hgap,int vgap):行数,列数,水平间距,垂直间距示例
import java.awt.*;
public class GridLayoutDemo extends Frame {
Button[] b = new Button[5];
public GridLayoutDemo(){
super("网格布局");
for(int i=0; i<b.length; i++){
b[i] = new Button("按钮"+i);
}
setLayout(new GridLayout(3,2));
add(b[0]);
add(b[1]);
add(b[2]);
add(b[3]);
add(b[4]);
pack();
setSize(300,100);
setLocation(100,200);
//setBounds(200.100,300,100);
setVisible(true);
}
public static void main(String[] args) {
new GridLayoutDemo();
}
}
pack():最紧凑的格式摆放
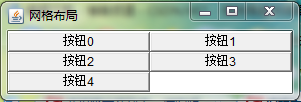
- CardLayout:卡片布局
- 布局效果:将多个组件在同一容器区域内交替显示,相当于多张卡片摞在一起,只有最上面的卡片是可见的。
- 构造方法
- public CardLayout()
- public CardLayout(int hgap, int vgap)
- 其他方法
- public void first(Container parent)—显示第一张卡片
- public void last(Container parent)—显示最后一张卡片
- public void previous(Container parent)—显示前一张卡片
- public void next(Container parent)—显示后一张卡片
- public void show(Container parent,String name)翻转到指定名称的组件,若不存在不发生操作
import java.awt.*;
public class CardLayoutDemo {
public static void main(String[] args) {
Frame f=new Frame("CardLayout Example");
CardLayout c1=new CardLayout();
f.setLayout(c1);
Label lbl[]=new Label[4];
for(int i=0;i<4;i++){
lbl[i]=new Label("第"+i+"页");
f.add(lbl[i],"card"+i);
}
while(true){
try{
Thread.sleep(1000);
}catch(InterruptedException e){
e.printStackTrace();
}
c1.next(f);
}
}
}
- GridBagLayout:动态矩形单元网格
分享到:
相关推荐
练习JAVA GUI设计和布局设计:编写一个窗体程序显示日历,只需要设计GUI,不需要事件处理。
java图形编程实例,非常好的javaGUI编程入门程序。
GUI java源码 多种例子 java大学课程
Java聊天软件chat_src用定制的GUI开发
java反编译工具,jd_guijava反编译工具,jd_guijava反编译工具,jd_guijava反编译工具,jd_guijava反编译工具,jd_guijava反编译工具,jd_guijava反编译工具,jd_guijava反编译工具,jd_guijava反编译工具,jd_gui...
java常见布局的例子,对gui编程很有帮助。
0216_JavaGUI[归类].pdf
java 图形界面的练习,适合新手练习使用
微盘下载的 SAP_GUI_730_JAVA_MAC
Windows操作系统自带的计算器是个很方便的小工具,利用Java的GUI编程,实现一个Java GUI计算器应用程序界面,窗口标题为“计算器”,窗口布局如下图所示,在此计算器应用程序中实现“+、-、*、/”运算操作
(1)分别运行工程两个包中的两个.java文件(UploadClient.java和UploadServer.java)分别会弹出“上传客服端”和“上传服务器”两个窗口。 (2)单击“上传服务器”窗口中的“启动服务..”按钮。 (3)单击“上传...
功能齐全的学籍管理系统,是利用javaGUI做的
这是一个java程序,希望大家好好学习哦!
java gui教程,涵盖了java gui的所有组件的创建及事件的创建说明
java GUI编程,可以编写出任意你想要的界面
初级学习者必备,开放的gui源代码,对于了解学习相关知识十分有用。
利用java实现的GUI编程,可以用来测试。
java 的简单应用,简单的JavaGUI 的应用
语言程序设计资料:JAVA__SE_应用程序设计__GUI程序设计-源代码.doc
除了加减乘除外还实现了许多的函数,比如sin,cos,求倒数,二十进制转换等,是我十多天的心血,程序非常长,也成功实现了设定的功能,有漂亮的用户界面,通过这个程序可以学到很多关于java GUI和计算器的知识